
#Python permutations how to
Moreover, understanding how to generate permutations manually using backtracking provides deeper insight into the problem and can be a starting point for solving more complex problems. The itertools.permutations() function is a powerful tool that can generate all permutations of any iterable. Generating all permutations of a set is a common task in programming and Python provides simple and effective tools to accomplish this task. It then swaps the elements back to restore the original list for the next iteration. It swaps each element with each element after it and then calls itself with the next index. In this example, generate_permutations() is a recursive function that generates all permutations of the given list of data.
#Python permutations generator
Here’s how you could implement a permutations generator function using backtracking: def generate_permutations(data, i, length): Backtracking is a general algorithm for finding all (or some) solutions to computational problems, particularly constraint satisfaction problems, which incrementally build candidates for the solutions, and abandons a candidate as soon as it determines that the candidate cannot possibly be extended to a valid solution.

To generate permutations manually, we can use a technique called backtracking. While the itertools module provides an easy and efficient way to generate permutations, it’s worth exploring how to generate permutations manually for educational purposes and for situations where you might need more control over the iteration. You can see that the itertools.permutations() function generates a sequence of tuples, where each tuple is a different permutation of the original set.
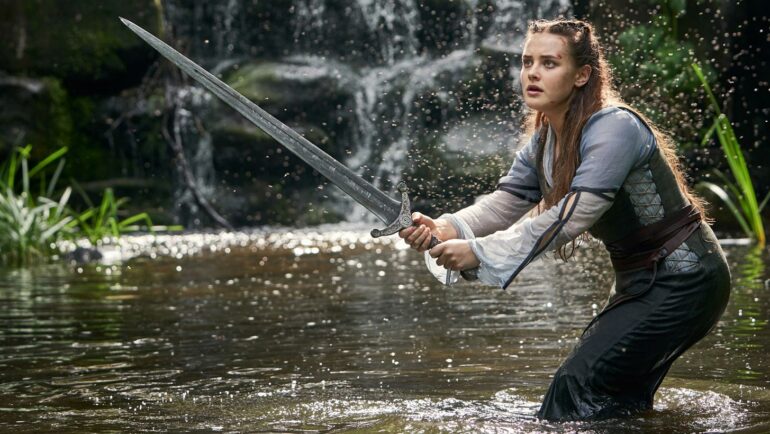
Permutations = itertools.permutations(numbers) Here’s a simple example: import itertools The function itertools.permutations() can be used to create a generator returning all permutations of a given set (or any other iterable). Among its many features, itertools has functions to generate all permutations of a set or list. Python’s itertools module is a collection of tools for handling iterators. In this article, we will explore how to generate all permutations of a set in Python, diving into the usage of the itertools module and how to implement permutation generation manually. Python, a high-level and interpreted programming language, provides several methods to generate permutations, particularly in its built-in module itertools. Generating permutations is a common task in programming, often done either to solve problems that require examining all possibilities or as a step in more complex algorithms.
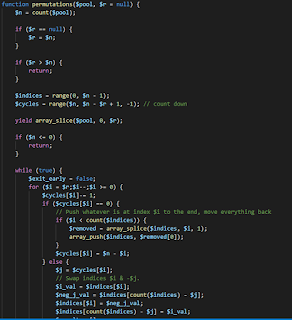
In computer science, the permutation of a set is, broadly speaking, an arrangement of its members into a sequence or linear order, or if the set is already ordered, a rearrangement of its elements.
